Hello, coding kittens and tech tigers! TheCodeCat here, whiskers twitching and tail high. Today, we’re going to refresh our knowledge on the SOLID principles. Whether you’re familiar with these concepts or preparing for an interview, this guide will help you recall the specifics and sharpen your coding claws.
SOLID Principles: The Five Catmandments of Codeville 🏰
In the grand realm of Codeville, the SOLID principles are revered as the five catmandments that uphold maintainable, flexible, and robust code. Envision these principles as the five whiskers of a cat, each one sensing a unique aspect of the coding environment:
- Single Responsibility Principle (SRP): The Solo Mouse Catcher 🐭
- Open-Closed Principle (OCP): The Cat Flap 🚪
- Liskov Substitution Principle (LSP): The Master of Disguise 🎭
- Interface Segregation Principle (ISP): The Fussy Feline 🍽️
- Dependency Inversion Principle (DIP): The Cat and the Laser Pointer 🐾
Let’s prowl into each of these principles.
Single Responsibility Principle (SRP): The Solo Mouse Catcher 🐭
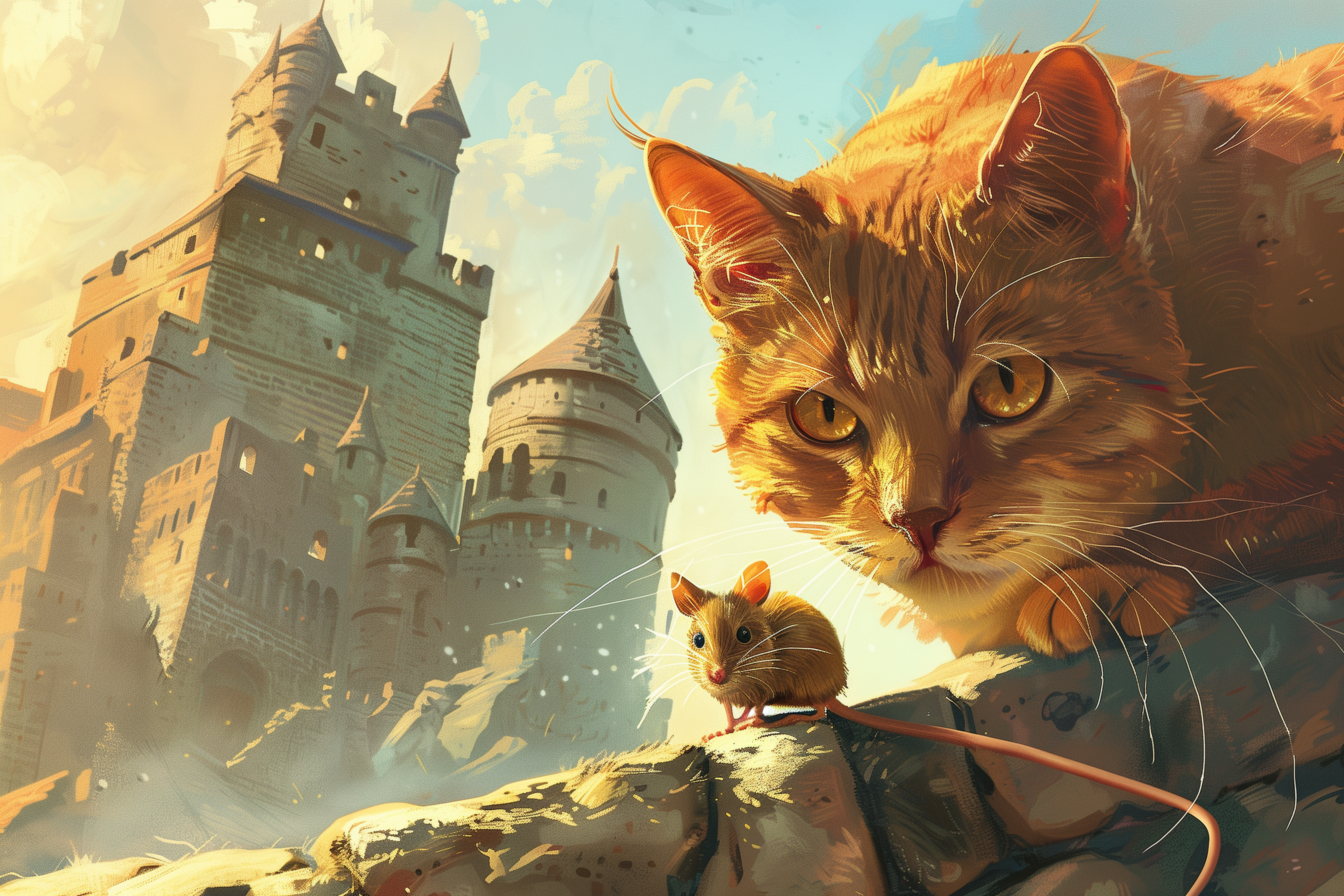
Picture a cat on a mission to catch a mouse. This cat is focused, determined, and has a single responsibility: to catch the mouse. Just like our mouse hunter, a class should only have one job or responsibility. By adhering to this principle, we can create a more robust and maintainable code, just like how our cat efficiently catches the mouse.
public class MouseHunter
{
public void Hunt() { /*...*/ }
}
In this example, the MouseHunter
class is like our solo mouse catcher, with a single responsibility: to hunt.
Open-Closed Principle (OCP): The Cat Flap 🚪
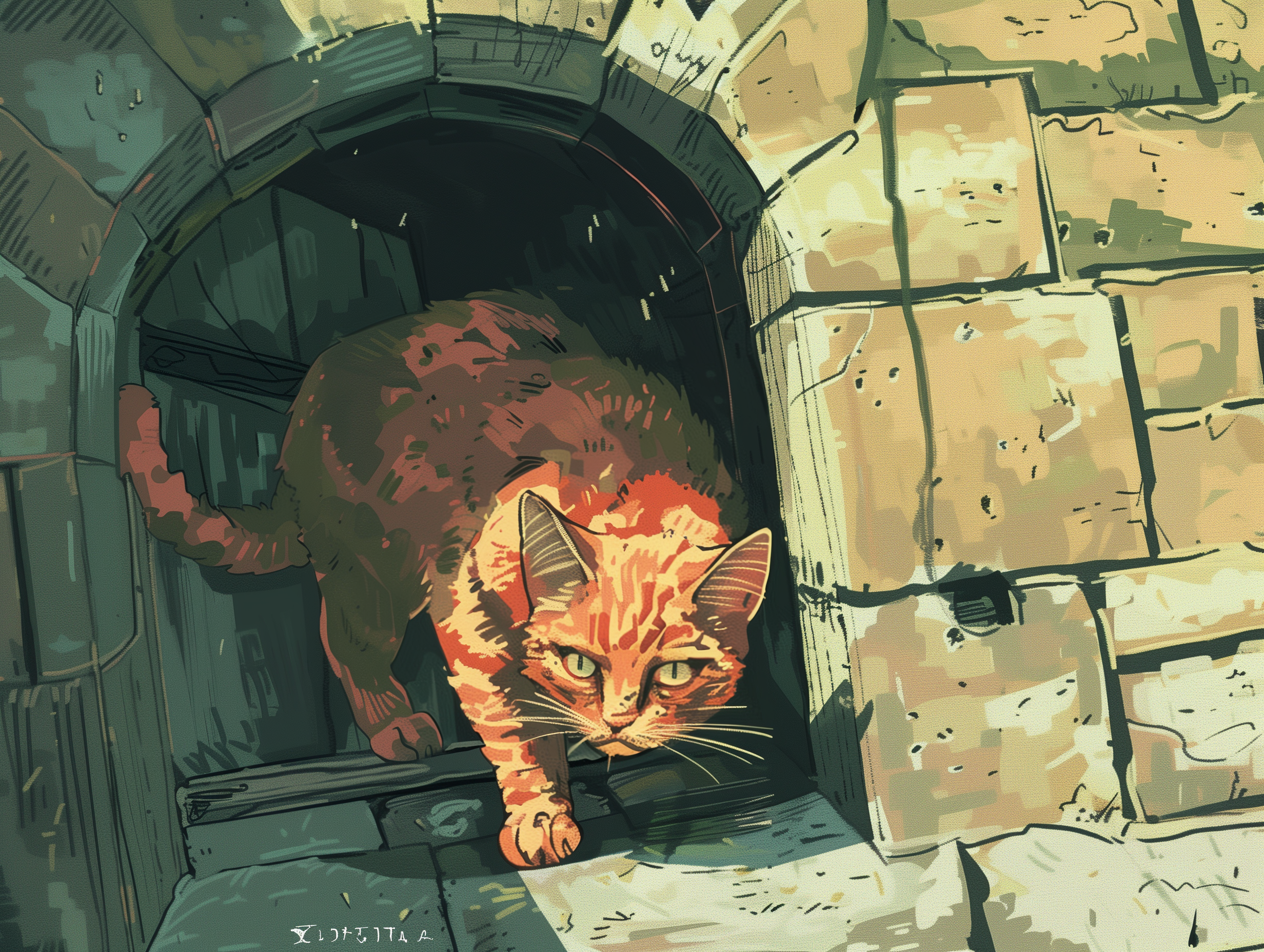
A cat flap is open for the cat to pass through, but closed to prevent unwanted guests. Similarly, classes should be open for extension but closed for modification.
public abstract class Cat
{
public abstract void Purr();
}
public class Tabby : Cat
{
public override void Purr() { /*...*/ }
}
public class Siamese : Cat
{
public override void Purr() { /*...*/ }
}
In this example, we can add new types of cats (like the cat flap opening for a new cat) without modifying the Cat
class or the Purr
method (like the cat flap closing to its original form).
Liskov Substitution Principle (LSP): The Master of Disguise 🎭
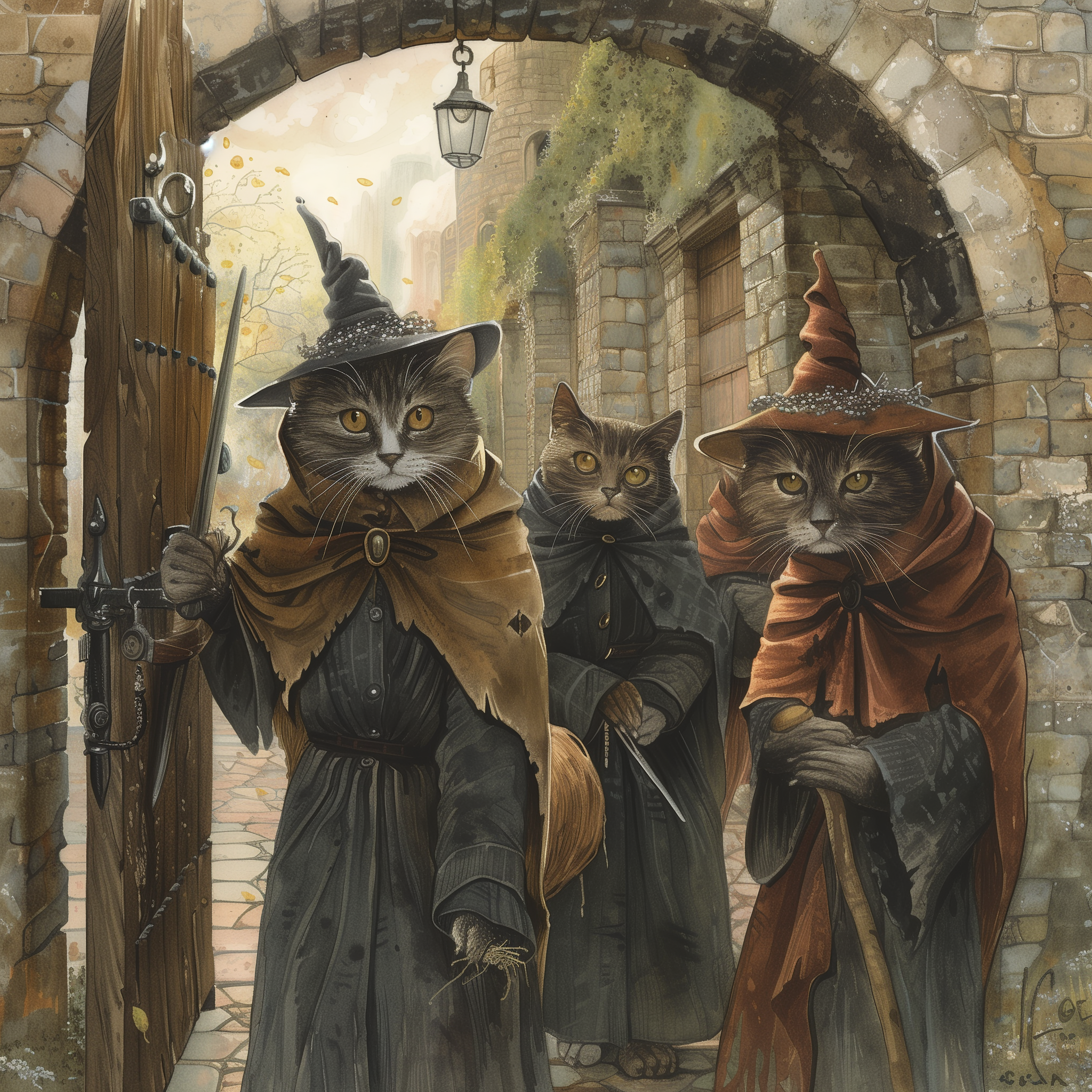
The Liskov Substitution Principle is like a cat with the magical ability to shape-shift into any other cat. If a program is using a base class, it should be able to use any of its subclasses without the program knowing it. In other words, the subclasses should be like shape-shifting cats that perfectly fit into the base class.
public class Cat
{
public virtual void Meow() { /*...*/ }
}
public class Persian : Cat { /*...*/ }
public class MaineCoon : Cat { /*...*/ }
In this example, Persian
and MaineCoon
are like shape-shifting cats that can seamlessly fit into the Cat
base class.
Interface Segregation Principle (ISP): The Fussy Feline 🍽️
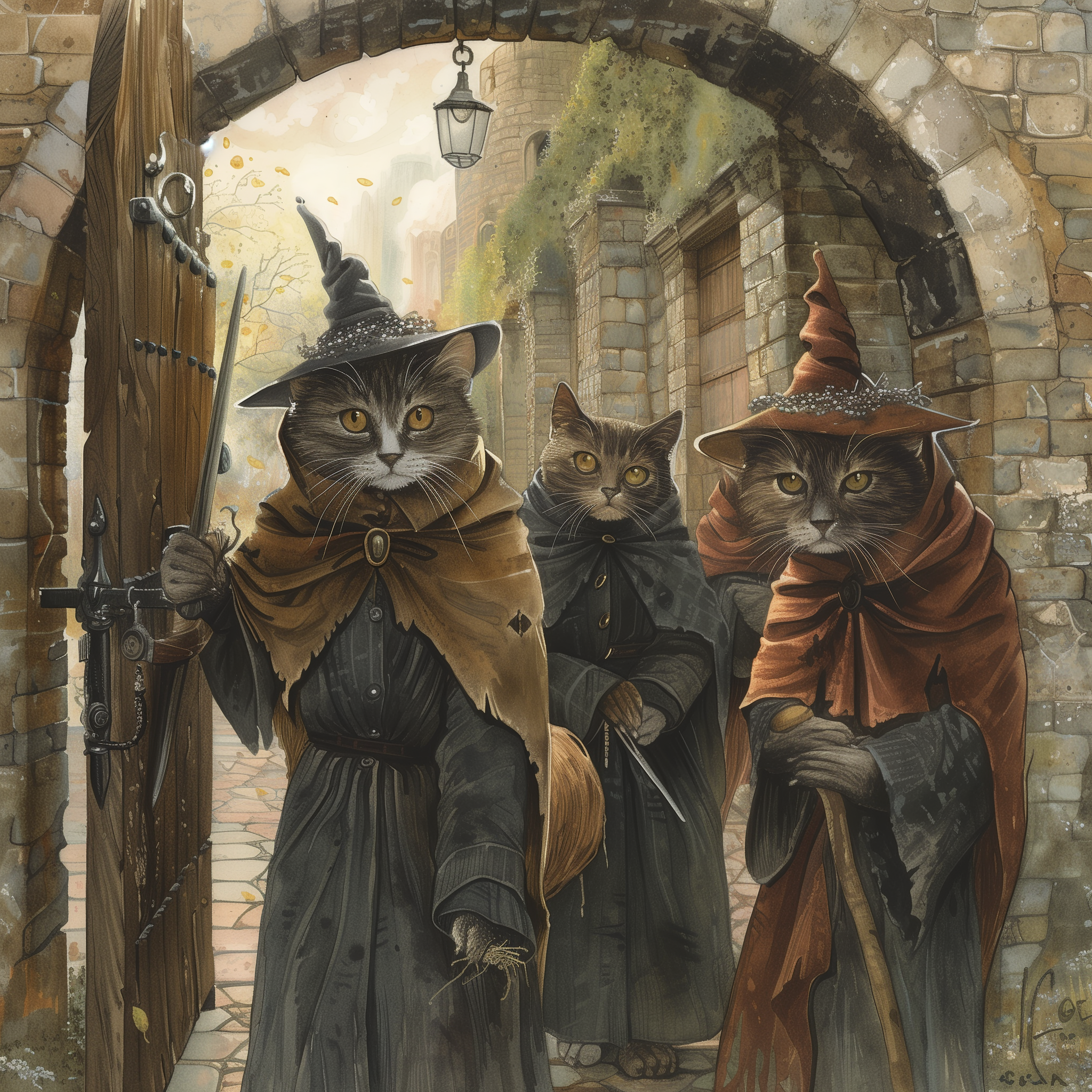
The Interface Segregation Principle is like a fussy feline at a buffet. The cat should not be forced to eat dishes they do not like. Similarly, a class should not have to implement methods it doesn’t use.
public interface ICat
{
void Purr();
void Eat();
}
public class RobotCat : ICat
{
public void Purr() { /*...*/ }
public void Eat()
{
throw new NotSupportedException();
}
}
In this example, RobotCat
is like a fussy feline who doesn’t eat, but it’s forced to implement the Eat
method.
Dependency Inversion Principle (DIP): The Cat and the Cat Tower 🏰
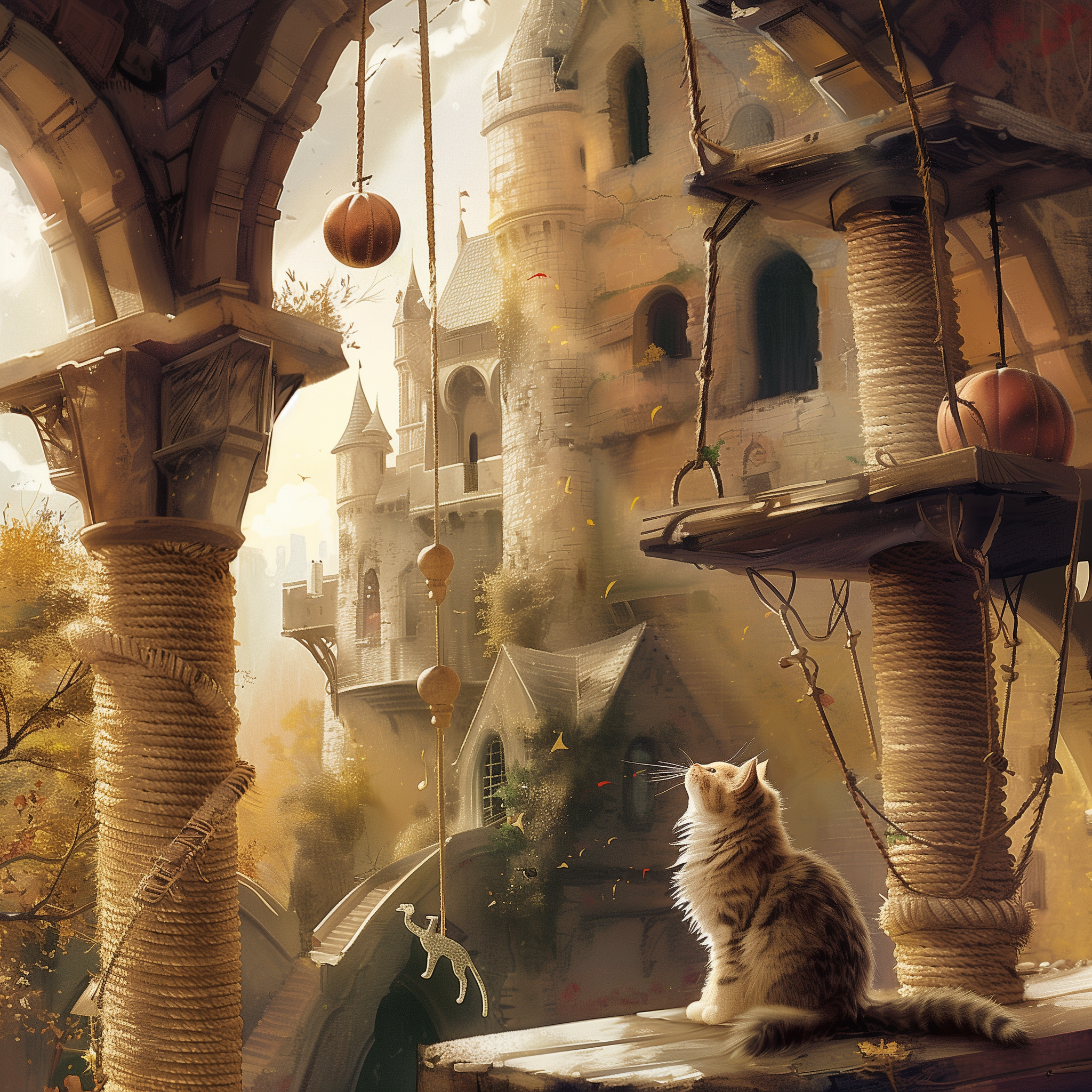
The Dependency Inversion Principle is like a cat playing on a cat tower. The cat interacts with different parts of the tower (like platforms, scratch posts, and hanging toys) without needing to know how they are attached or supported. Similarly, high-level modules (like the cat) should not depend on low-level modules (like the specific parts of the cat tower). Instead, both should depend on abstractions (like the general concept of a cat tower).
public interface ICatTower
{
void Climb();
void Scratch();
}
public class SimpleCatTower : ICatTower
{
public void Climb() { /*...*/ }
public void Scratch() { /*...*/ }
}
public class FancyCatTower : ICatTower
{
public void Climb() { /*...*/ }
public void Scratch() { /*...*/ }
}
public class Cat
{
private ICatTower _catTower;
public Cat(ICatTower catTower)
{
_catTower = catTower;
}
public void UseCatTower()
{
_catTower.Climb();
_catTower.Scratch();
}
}
In this example, the Cat interacts with any type of cat tower through the ICatTower interface, just like a cat plays on various parts of a cat tower without knowing how they are built. This design ensures that high-level modules (like Cat) depend on abstractions (ICatTower), not on concrete implementations (like SimpleCatTower or FancyCatTower), adhering to the DIP.
By focusing on abstractions, our code becomes more flexible and easier to maintain, just like how a cat can enjoy any type of cat tower without needing to understand its construction.
SOLID Exercises: The Cat’s Playground 🐾
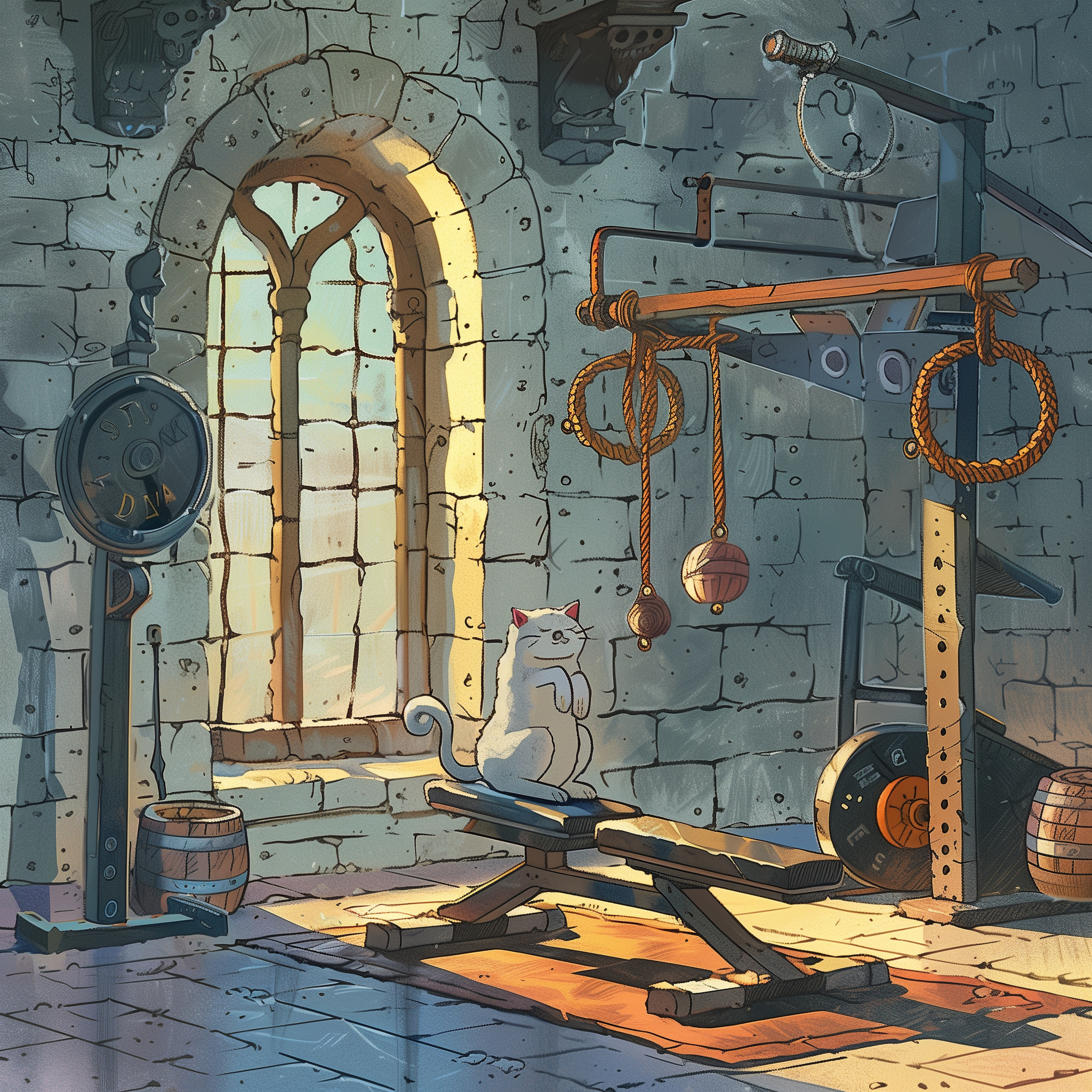
Now that we’ve prowled through the alleyways of SOLID principles, it’s time to put your knowledge to the test. Here are some playful exercises for you to flex your coding claws on:
-
Single Responsibility Principle (SRP): Consider a
Cat
class with methodsPurr()
,Scratch()
, andSleep()
. The SRP states that a class should only have one reason to change. Does theCat
class adhere to this principle? If not, refactor the class to ensure it only has one responsibility.Exercise: Create a
Cat
class with the methodsPurr()
,Scratch()
, andSleep()
. Then, refactor the class to adhere to the Single Responsibility Principle. -
Open-Closed Principle (OCP): Now, let’s consider the
CatFood
class with methodsDryFood()
,WetFood()
, andTreats()
. The OCP states that software entities should be open for extension, but closed for modification. Does theCatFood
class adhere to this principle? If not, refactor the class to ensure it does.Exercise: Create a
CatFood
class with the methodsDryFood()
,WetFood()
, andTreats()
. Then, refactor the class to adhere to the Open-Closed Principle. -
Liskov Substitution Principle (LSP): Imagine you have a
Cat
base class andPersian
andMaineCoon
subclasses. The LSP states that if a program is using a base class, it should be able to use any of its subclasses without the program knowing it. Does your code adhere to this principle? If not, refactor it to ensure it does.Exercise: Create a
Cat
base class andPersian
andMaineCoon
subclasses. Then, refactor the classes to adhere to the Liskov Substitution Principle. -
Interface Segregation Principle (ISP): Consider a
Cat
interface with methodsPurr()
,Scratch()
,Sleep()
, andEat()
. The ISP states that no client should be forced to depend on interfaces they do not use. Does theCat
interface adhere to this principle? If not, refactor it to ensure it does.Exercise: Create a
Cat
interface with the methodsPurr()
,Scratch()
,Sleep()
, andEat()
. Then, refactor the interface to adhere to the Interface Segregation Principle. -
Dependency Inversion Principle (DIP): Finally, consider a
CatOwner
class that depends on aPersian
cat class. The DIP states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Does theCatOwner
class adhere to this principle? If not, refactor it to ensure it does.Exercise: Create a
CatOwner
class that depends on aPersian
cat class. Then, refactor the class to adhere to the Dependency Inversion Principle.
Remember, the goal of these exercises is to help you understand and apply the SOLID principles in your coding journey. Happy coding! 🐾
Typical Interview Questions and Answers about SOLID: The Cat’s Quiz 🎓
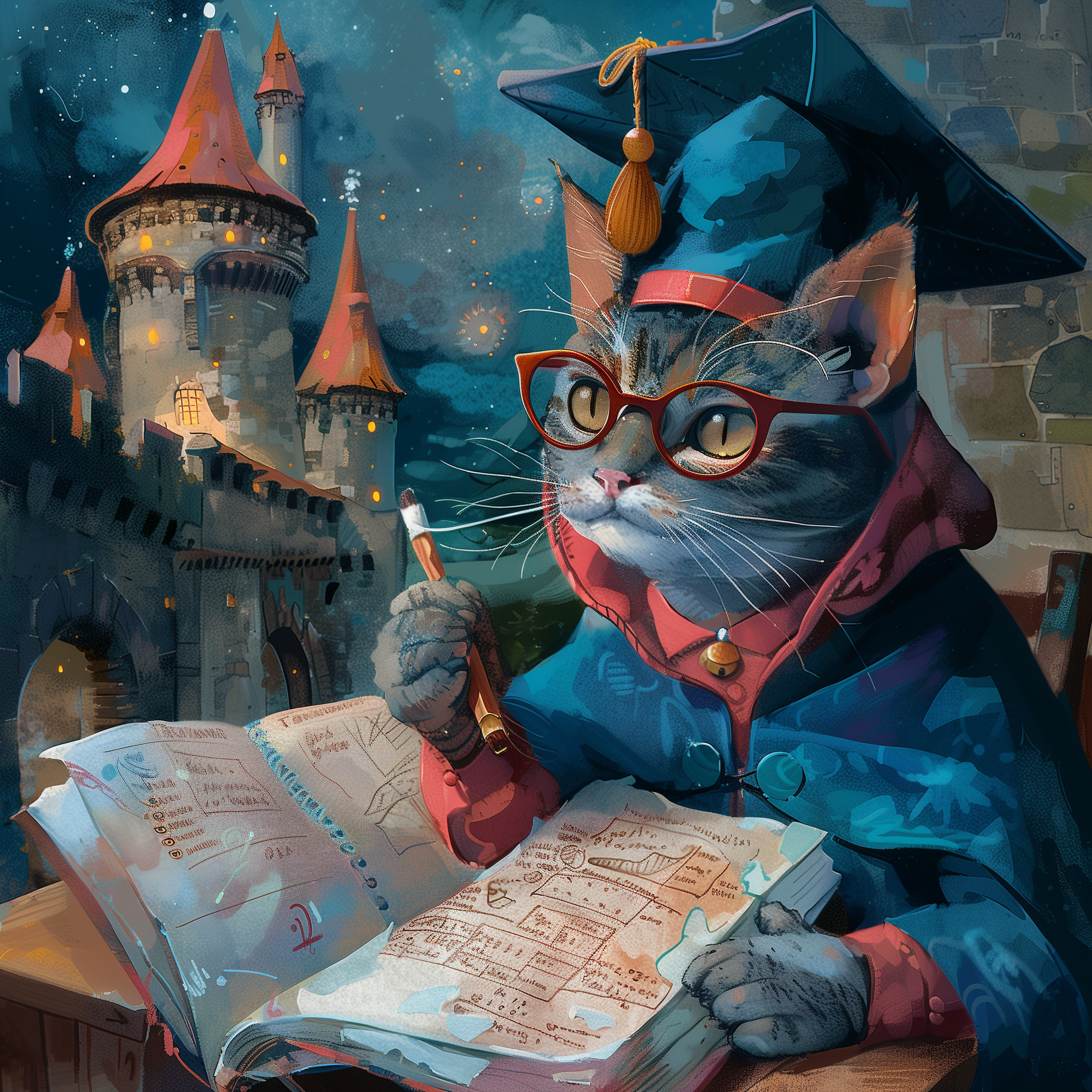
As you prepare for your interview, here are some typical questions and answers about SOLID principles:
Q: Can you give an example of the Single Responsibility Principle?
A: The Single Responsibility Principle states that a class should have only one reason to change. For example, a Cat
class should only manage the properties and behaviors of a cat, not the database operations related to the cat.
Q: How does the Open-Closed Principle improve the maintainability of code? A: The Open-Closed Principle improves maintainability by allowing new functionality to be added without changing existing code. This reduces the risk of introducing new bugs in existing code.
Q: Can you explain the Liskov Substitution Principle with an example?
A: The Liskov Substitution Principle states that if a program is using a base class, it should be able to use any of its subclasses without the program knowing it. For example, if a program is using a Cat
class, it should be able to use a Persian
or MaineCoon
subclass without the program knowing it.
Q: How does the Interface Segregation Principle affect the design of interfaces? A: The Interface Segregation Principle affects the design of interfaces by ensuring that clients are not forced to depend on interfaces they do not use. This leads to smaller, more focused interfaces.
Q: Can you give an example of the Dependency Inversion Principle?
A: The Dependency Inversion Principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. For example, a CatOwner
class should depend on the ICat
interface, not the Persian
cat class.
Conclusion: The End of the Catwalk 🐈
The SOLID principles are the five pillars that uphold the kingdom of object-oriented programming. Understanding and applying these principles can help you write code that is robust, maintainable, and flexible. As you prepare for your interview, remember that these principles are not just rules to follow, but tools to help you design better systems.
Remember, the future of coding is not just in the hands of its designers, but also in the hands of its users. Your understanding and application of these principles can help shape the future of programming, making it more powerful, more flexible, and more suited to the needs of developers. So don’t hesitate to dive deeper into these principles and apply them in your coding journey.
And so, our tail… I mean, tale comes to an end. But remember, every end is just a new beginning. So, stay curious, stay bold, and as always, keep your whiskers in the wind and your paws on the keyboard! 🐾
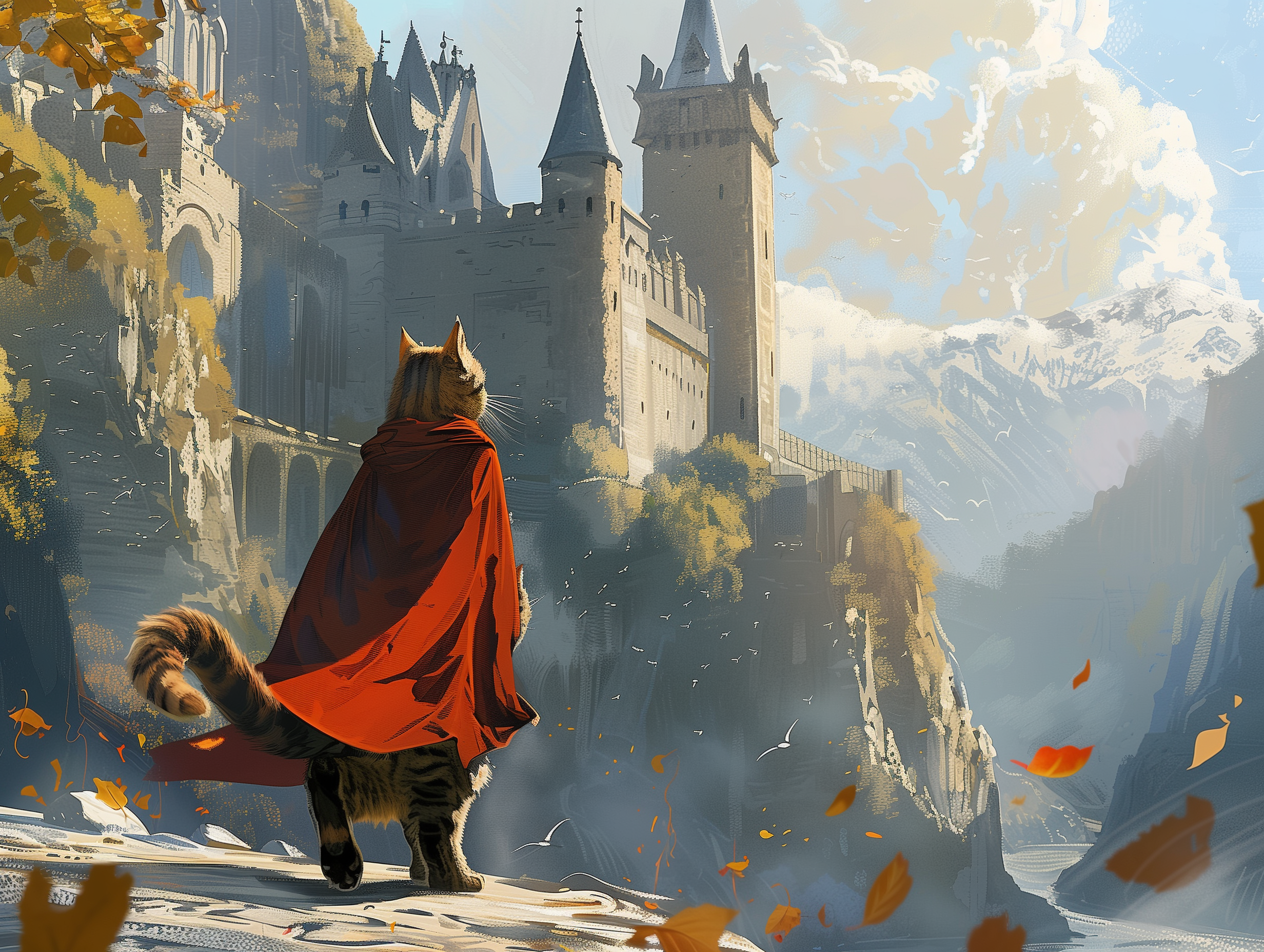